API
When to use :
This tool created for customers that want to upload emails list and download results in automatic mode. For example if you using bulk verification tool you need to: login to our service, open upload form, choose file, wait until file will be finished, download results.
But this API allow to do this work for your software in fully automatic mode without login to site.
How it works :
- your software uploading file with emails to our API and getting {file_id} as result.
- your software checking status of file by {file_id} (once per 10 minutes for example)
- when status of file is "finished", your software downloading reports
Upload file with API :
There is link for file upload:
https://app.mycleanlist.com/api/bulk_email_verify.php?key=USERKEY&filename={filename}&unique={unique}
{filename} - name of your file, required
{unique} - for remove duplicates use "yes" (but it taking additional time), leave it empty on other case, optional
Example of PHP code:
$settings = array( 'filename' => $cFile->postname, 'fileData' => $cFile );
$url = "https://app.mycleanlist.com/api/bulk_email_verify.php?key=USERKEY&filename=my_emails.txt";
$curl = curl_init($url);
curl_setopt($curl, CURLOPT_TIMEOUT, 30);
curl_setopt($curl, CURLOPT_POST, 1);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl, CURLOPT_HTTPHEADER, array( 'Content-Type: multipart/form-data' ) );
curl_setopt($curl, CURLOPT_POSTFIELDS, $settings);
curl_setopt($curl, CURLOPT_INFILESIZE, $fileSize);
$response = curl_exec($curl);
curl_close($curl);
Example of output when success :
97381
Example of output when error happens :
error_no_file_uploaded
Output result is error status, or FILE ID that you can use for access file information.
Full errors list :
- error_no_file_uploaded - no file was submitted to API
- error_too_many_files - more than one file submitted or more than 100 active files
- error_upload - file uploaded as broken
- error_no_filename - filename is empty
- error_disallowed - your balance is $0 or key is wrong
- error_file_is_empty - file is empty
- error_internal - error inside our script happens
Get file info with API :
There is link that you need to request:
https://app.mycleanlist.com/api/file_info.php?key=USERKEY&ext=2&fileid={fileid}
{id} -FILE ID of already uploaded file, required
Example of PHP code:
$file_id = 97381
$url = 'https://app.mycleanlist.com/api/file_info.php?key=USERKEY&ext=2&fileid='.$file_id;
$string = file_get_contents($url); //execute request to api
list($file_id, $filename, $unique, $lines, $processed, $ok, $invalid ,$unknown, $reverify, $status, $timestamp, $link1, $link2, $link3) = explode('|',$string);
Example of output for file that in progress :
97381| my_emails.txt| no| 100| 50| 30| 15| 5| progress| 1386422072|||
Example of output for file that already finished :
97381| my_emails.txt| no| 100| 100| 60| 20| 10| finished| 1386422072 | https://app.mycleanlist.com/download_emails.php?fid=97381&type=1| https://app.mycleanlist.com/download_emails.php?fid=97381&type=2| https://app.mycleanlist.com/download_emails.php?fid=97381&type=3
Example of output when file with ID 97381 not exists :
error_file_not_exists
Output result is error status or string with information about file.
Full errors list:
- error_id_missing - FILE ID is missing in your request
- error_file_not_exists - FILE ID is not exists on your account
- error_disallowed - your balance is $0 or key is wrong
- error_internal - error inside our script happens
String with information:
{file_id}|{filename}|{unique}|{lines}|{lines_processed}|{ok}|{invalid}|{unknown}|{status}|{timestamp}|{link_all}|{link_ok}|{link_detail}
- {file_id} - the same FILE ID as you use for request "file info"
- {filename} - name of file (same as you use during file upload)
- {unique} - "yes" or "no", remove duplicates option (same as you use during file upload)
- {lines} - total number of parsed emails in file
- {processed} -number of already processed emails
- {ok} -number of valid emails at that moment
- {invalid} -number of invalid emails at that moment
- {unknown} -number of "unknown" emails at that moment
- {reverify} -number of emails with "unknown" status that will be re-verified
- {status} -file status, it can be: new, parsing, incorrect , waiting , progress , suspended, canceled, finished
- {timestamp} -date-time when you upload file (in unix format)
- {link_all} -link to report "all", if link is empty - file is not finished yet.
- {link_ok} -link to report "ok", if link is empty - file is not finished yet.
- {link_detail} -link to report "detail", if link is empty - file is not finished yet.
Get files list with API :
There is link that you need to request :
https://app.mycleanlist.com/api/get_file_list.php?key=USERKEY
Example of PHP code :
$url = https://app.mycleanlist.com/api/get_file_list.php?key=USERKEY
$string = file_get_contents($url);
$files_list = explode('|',$string);
Example of output if three files was uploaded :
97381|97382|92382
Example of output if no files was uploaded :
error_no_files
Output result is error status or string with FILE ID's.
Full errors list :
- error_no_files -no files was uploaded to your account
- error_disallowed -your balance is $0 or key is wrong
- error_internal -error inside our script happens
Get All Status of emails with API :
There is link that you need to request:
https://app.mycleanlist.com/api/file_download_status.php?key=USERKEY&fileid={fileid}
Example of output :
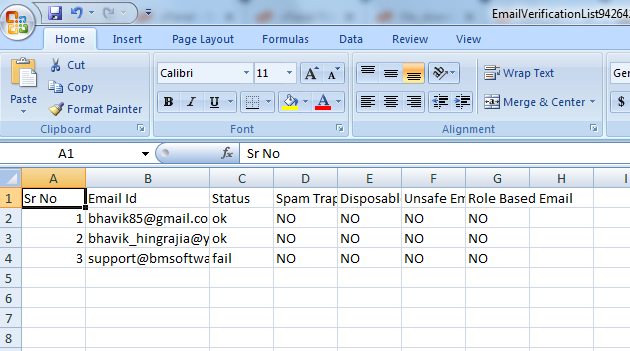
Cancel file (verification will be stopped and results will be ready soon) :
There is link that you need to request :
https://app.mycleanlist.com/api/file_upload_cancel.php?key=USERKEY&fileid={fileid}
Example of PHP code:
$file_id = 97381
$url = 'https://app.mycleanlist.com/api/file_upload_cancel.php?key=USERKEY&fileid='.$file_id;
$result = file_get_contents($url);
Example of output if file was canceled :
done
Example of output if file not found :
error_file_not_exists
Output result is error status or string 'done'.
Full errors list:
- error_id_missing -you forget to send FILE ID &id={file_id}
- error_disallowed -your balance is $0 or key is wrong
- error_file_not_exists -FILE ID is not exists on your account
- file_status_update_error -status of file was not updated for some reason
- wrong_file_status_for_cancel -you can't cancel file if it in parsing or already canceled/finished